In the last tutorial, we saw together how to set up the general architecture for .NET Core Web API. If you haven’t read the article, here’s the link.
In this new tutorial, we ‘ll see how to:
- Configure the settings file and put the database connection string.
- Call UsersManager in UsersController.
- Use Postman tool to send different type of HTTP requests.
You can fine more details step by step in my video on YouTube or get all the code source on GitHub.
Set up settings file
First of all, open your appsettings.json file and add the connection string to you local SQLServer database. The string look like:
"ConnectionStrings": {
"RefreshTokenDb": "Data Source=(LocalDb)\\MSSQLLocalDB;Initial Catalog=RefreshToekn;Integrated Security=SSPI;"
},
Note that you need to put this json text in appsettings.Development.json, because by default you will run the application on development environment.
After that, in the Startup.cs file, inject the database context using the settings service. This is very important to configure your application because in real project, you have 2 or 3 environments. So each one has its own configuration (Database, url ….).
public void ConfigureServices(IServiceCollection services)
{
var appSettingsSection = Configuration.GetSection("AppSettings");
var appSettings = appSettingsSection.Get<AppSettings>();
services.AddControllers();
services.AddDbContext<RefreshTokenContext>(option =>
{
option.UseSqlServer(Configuration.GetConnectionString("RefreshTokenDb"));
});
services.AddScoped<IUnitOfWork, UnitOfWork>();
services.AddScoped<IUsersRepository, UsersRepository>();
services.AddScoped<IUsersManager, UsersManager>();
}
Finally, in the UsersController, we need to inject the UsersManager dependency and use it in the different methods (Get, update…..).
So the UsersController will look like:
[Route("api/[controller]")]
[ApiController]
public class UsersController : ControllerBase
{
private readonly IUsersManager usersManager;
public UsersController(IUsersManager usersManager)
{
this.usersManager = usersManager;
}
[HttpGet("GetUsers")]
public IActionResult GetUsers()
{
return Ok(usersManager.GetAllUsers());
}
[HttpPost("AddUser")]
public IActionResult AddUser([FromBody] UserModel userModel)
{
var userID = usersManager.Insert(userModel);
return Ok(userID);
}
}
Postman
Postman is a free tool through which we can test API end point. We can run all type of HTTP requests (Get, Put, Post….). Let’s see together how it works.
First of all, you have to install it from the official web site. After that, run your Web API in debug mode.
Like we run the web API on localhost, you have to turn off the SSL verification under Settings as shown in the image below:
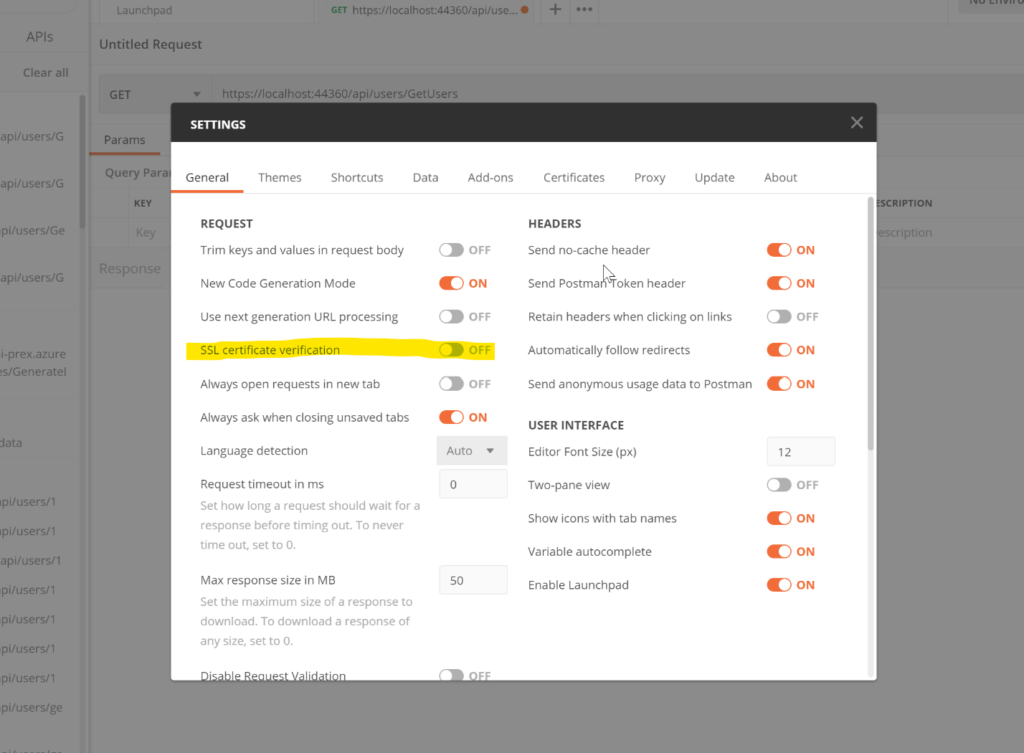
Now, let’s insert new User in the database. That’s why you have to put your url to the inset action and change the type of HTTP request to POST. Don’t forget to put your data to insert in the body of your request.
Click on the Send button and the response of your request is the ID of the inserted user.
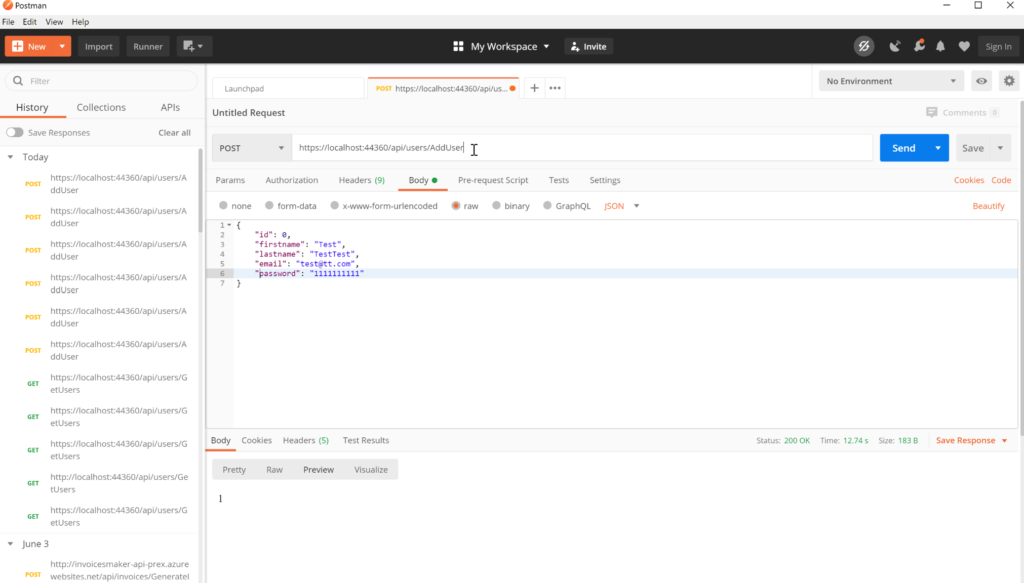
Finally, you can run the GetAll actions and if we have a records in the database we will see all the users. In my case I have only one user. You can enjoy creating more users.
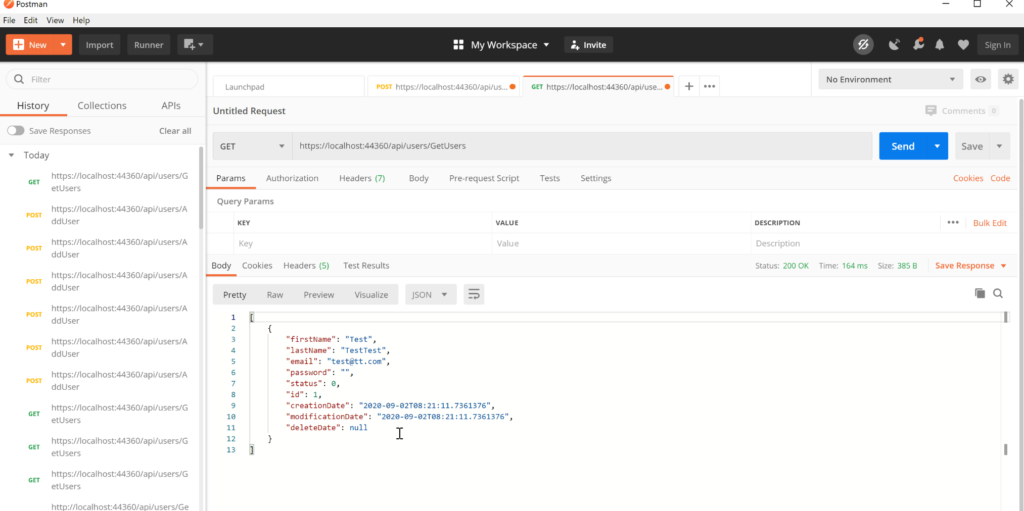
That’s all for this tutorial, next time we’ll see how to make JWT token authentication.
Follow Me For Updates
Subscribe to my YouTube channel or follow me on Twitter or GitHub to be notified when I post new content.
It’s awesome designed for me to have a web page, which
is useful designed for my knowledge. thanks admin
Good post! We will be linking to this great content on our site.