In this new tutorial series, we will see how to develop an E-commerce application, using Xamarin forms Prism template. In order to install Prism template, I invite you to check my first Xamarin Forms – Prism.
The code is available on GitHub.
You can also see the part one of this tutorial on this link.
Add ProductDetailsPage
Let’s begin by adding new tag called CarouselView through which we will display the different product images. For more details you can see my post for CarouselView .
Bind the ItemsSource to ProductImagesList that will be defined in the view model.
So you code will look like this:
<Grid RowDefinitions="3.8*,30,50,auto,*"
Margin="5"
ColumnDefinitions="*,*">
<CarouselView ItemsSource="{Binding ProductImagesList}"
Margin="0,5"
Grid.ColumnSpan="2"
IndicatorView="IndicatorView">
<CarouselView.ItemTemplate>
<DataTemplate>
<StackLayout>
<Frame HasShadow="True"
BorderColor="DarkGray"
CornerRadius="5"
HorizontalOptions="Center"
Padding="5"
VerticalOptions="Center">
<Image Source="{Binding }"
Aspect="AspectFill"/>
</Frame>
</StackLayout>
</DataTemplate>
</CarouselView.ItemTemplate>
</CarouselView>
</Grid>
Like in HTML, we can add also Carousel items indicator by using the IndicatorView component.
<IndicatorView x:Name="IndicatorView"
Grid.ColumnSpan="2"
Grid.Row="1"
IndicatorColor="LightGray"
SelectedIndicatorColor="DarkGreen"
HorizontalOptions="Center"/>
Finally, in this view, add product label, price and buy button to add product to the basket. Note that styles were already defined in App.xaml file.
<Label Grid.Row="2"
Text="{Binding ProductName}"
Style="{StaticResource ProductTitleLabelStyle}"/>
<Frame Grid.Row="2"
Grid.Column="1"
CornerRadius="5"
HasShadow="True"
Margin="0"
Padding="0"
BackgroundColor="{StaticResource StylishGrayColor}"
WidthRequest="100"
HorizontalOptions="Center"
BorderColor="Gray">
<Label Text="{Binding Price, StringFormat='{0:C2}'}"
Style="{StaticResource ProductPriceLabelStyle}"/>
</Frame>
<Label Grid.Row="3"
Grid.ColumnSpan="2"
Style="{StaticResource ProductDescriptionLabelStyle}"
Text="Premium dress with embroidery and lace Composition: 100% POLYESTER"/>
<Frame Grid.Row="4"
Grid.ColumnSpan="2"
Style="{StaticResource ButtonFrameStyle}">
<Button Style="{StaticResource ButtonStyle}"
Text="Buy"/>
</Frame>
App.xaml file:
<Style x:Key="ProductTitleLabelStyle" TargetType="Label">
<Setter Property="TextColor" Value="{StaticResource BlackColor}"/>
<Setter Property="FontSize" Value="18" />
<Setter Property="VerticalOptions" Value="Center"/>
<Setter Property="HorizontalOptions" Value="Center"/>
</Style>
<Style x:Key="ProductPriceLabelStyle" TargetType="Label">
<Setter Property="VerticalOptions" Value="Center"/>
<Setter Property="HorizontalOptions" Value="Center"/>
<Setter Property="FontSize" Value="15" />
<Setter Property="TextColor" Value="{StaticResource WhiteColor}" />
</Style>
<Style x:Key="ProductDescriptionLabelStyle" TargetType="Label" BasedOn="{StaticResource ProductTitleLabelStyle}">
<Setter Property="FontSize" Value="15" />
</Style>
In the View model, and like others, define 2 regions Properties and commands.
Now, define ProductImagesList, ProductName and Price. Inject the products service like a dependency in the ViewModel ‘s constructor:
#region Properties
private readonly IProductsService productsService;
private IEnumerable<string> productImagesList;
public IEnumerable<string> ProductImagesList
{
get => productImagesList;
set => SetProperty(ref productImagesList, value);
}
private string productName;
public string ProductName
{
get => productName;
set => SetProperty(ref productName, value);
}
private float price;
public float Price
{
get => price;
set => SetProperty(ref price, value);
}
#endregion
In the products page, we have already passed the serialized product object. So, we get it from the navigation parameter, and define the different properties like ProductName, ProductImagesList and Price.
The right place to make this initialization is to override the method Initialize .
public override void Initialize(INavigationParameters parameters)
{
ProductModel productModel = JsonConvert.DeserializeObject<ProductModel>(parameters.GetValue<string>("Product"));
Title = productModel.Name;
ProductImagesList = productsService.GetProductsImages(productModel.ID);
ProductName = productModel.Name;
Price = productModel.Price;
}
Eventually, in products add a new method called GetProductsImages to get product images list by product ID. In our case, I did a stub. you can get it on GitHub.
Run the application and you will get all your products with a nice Carousel View.
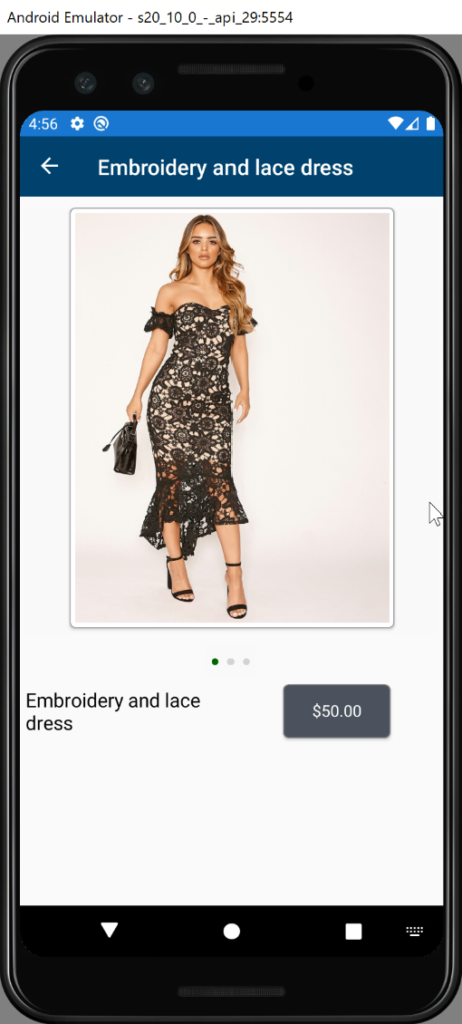
In this last part of post, you’re going to add the product size list, quantity and the buy button.
So you Product details page code will look like this:
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:prism="http://prismlibrary.com"
prism:ViewModelLocator.AutowireViewModel="True"
x:Class="Shopping.Views.ProductDetailsPage"
Title="{Binding Title}">
<ScrollView>
<Grid RowDefinitions="400,30,50,auto,auto,80,80"
Margin="5"
ColumnDefinitions="*,*">
<CarouselView ItemsSource="{Binding ProductImagesList}"
Margin="0,5"
Grid.ColumnSpan="2"
IndicatorView="IndicatorView">
<CarouselView.ItemTemplate>
<DataTemplate>
<StackLayout>
<Frame HasShadow="True"
BorderColor="DarkGray"
CornerRadius="5"
HorizontalOptions="Center"
Padding="5"
VerticalOptions="Center">
<Image Source="{Binding }"
Aspect="AspectFill"/>
</Frame>
</StackLayout>
</DataTemplate>
</CarouselView.ItemTemplate>
</CarouselView>
<IndicatorView x:Name="IndicatorView"
Grid.ColumnSpan="2"
Grid.Row="1"
IndicatorColor="LightGray"
SelectedIndicatorColor="DarkGreen"
HorizontalOptions="Center"/>
<Label Grid.Row="2"
Margin="20,0,0,0"
Text="{Binding ProductName}"
Style="{StaticResource ProductTitleLabelStyle}"/>
<Frame Grid.Row="2"
Grid.Column="1"
CornerRadius="5"
HasShadow="True"
Margin="0"
Padding="0"
BackgroundColor="{StaticResource StylishGrayColor}"
WidthRequest="100"
HorizontalOptions="Center"
BorderColor="Gray">
<Label Text="{Binding Price, StringFormat='{0:C2}'}"
Style="{StaticResource ProductPriceLabelStyle}"/>
</Frame>
<Label Grid.Row="3"
Grid.ColumnSpan="2"
Margin="20,10,10,10"
Style="{StaticResource ProductDescriptionLabelStyle}"
Text="Premium dress with embroidery and lace Composition: 100% POLYESTER"/>
<StackLayout Grid.Row="4"
Grid.ColumnSpan="2"
Margin="20,0,0,0"
Orientation="Horizontal">
<Label Text="Size:"
TextColor="{StaticResource BlackColor}"
VerticalTextAlignment="Center"/>
<Picker ItemsSource="{Binding SizeList}" WidthRequest="40" HorizontalOptions="Center"
SelectedItem="{Binding SelectedSize}" />
</StackLayout>
<StackLayout Grid.Row="5"
Grid.ColumnSpan="2"
Orientation="Horizontal"
Margin="20">
<Label Text="Quantity:"
TextColor="{StaticResource BlackColor}"
VerticalTextAlignment="Center" />
<Label Text="{Binding Quantity}"
TextColor="{StaticResource BlackColor}"
VerticalTextAlignment="Center"/>
<Button Text="-"
Command="{Binding DecreaseQuantityCommand}"
VerticalOptions="Center"
WidthRequest="40"/>
<Button Text="+"
Command="{Binding IncreaseQuantityCommand }"
VerticalOptions="Center"
WidthRequest="40"/>
</StackLayout>
<Frame Grid.Row="6"
Grid.ColumnSpan="2"
Style="{StaticResource ButtonFrameStyle}">
<Button Style="{StaticResource ButtonStyle}"
Text="Buy"/>
</Frame>
</Grid>
</ScrollView>
</ContentPage>
<script async src="https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js"></script>
<!-- Middle In Article -->
<ins class="adsbygoogle"
style="display:block"
data-ad-client="ca-pub-3326886530249247"
data-ad-slot="7484259302"
data-ad-format="auto"
data-full-width-responsive="true"></ins>
<script>
(adsbygoogle = window.adsbygoogle || []).push({});
</script>
In ViewModel, define the size list, selected size, quantity and the different commands like increase or decrease the quantity.
using Newtonsoft.Json;
using Prism.Commands;
using Prism.Navigation;
using Shopping.Models.Models;
using Shopping.Services.Interfaces;
using System.Collections.Generic;
using System.Windows.Input;
namespace Shopping.ViewModels
{
public class ProductDetailsPageViewModel : ViewModelBase
{
#region Properties
private readonly IProductsService productsService;
private IEnumerable<string> productImagesList;
public IEnumerable<string> ProductImagesList
{
get => productImagesList;
set => SetProperty(ref productImagesList, value);
}
private string productName;
public string ProductName
{
get => productName;
set => SetProperty(ref productName, value);
}
private float price;
public float Price
{
get => price;
set => SetProperty(ref price, value);
}
private int quantity;
public int Quantity
{
get => quantity;
set=> SetProperty(ref quantity, value);
}
private IEnumerable<string> sizeList;
public IEnumerable<string> SizeList
{
get => sizeList;
set => SetProperty(ref sizeList, value);
}
private string selectedSize;
public string SelectedSize
{
get => selectedSize;
set => SetProperty(ref selectedSize, value);
}
#endregion
#region Commands
public ICommand IncreaseQuantityCommand { get; set; }
public ICommand DecreaseQuantityCommand { get; set; }
#endregion
public ProductDetailsPageViewModel(INavigationService navigationService, IProductsService productsService)
: base(navigationService)
{
this.productsService = productsService;
IncreaseQuantityCommand = new DelegateCommand(IncreaseQuantity);
DecreaseQuantityCommand = new DelegateCommand(DecreaseQuantity);
}
private void DecreaseQuantity()
{
if(Quantity >= 2)
{
Quantity--;
}
}
private void IncreaseQuantity()
{
Quantity++;
}
public override void Initialize(INavigationParameters parameters)
{
ProductModel productModel = JsonConvert.DeserializeObject<ProductModel>(parameters.GetValue<string>("Product"));
Title = productModel.Name;
ProductImagesList = productsService.GetProductsImages(productModel.ID);
ProductName = productModel.Name;
Price = productModel.Price;
// Fake data
SizeList = new List<string>() { "S", "M", "L" };
Quantity = 1;
}
}
}
Finally, run the application and enjoy the shopping in your application.
Follow Me For Updates
Subscribe to my YouTube channel or follow me on Twitter or GitHub to be notified when I post new content.
can you give a complete code for this application. I am Stuck
The code is available on GitHub.