Hello, every body in this new tutorial of Xamarin Forms. In this part, you will learn how to make a basic local notification.
NotificationManager
First of all, add a new button in MainPage.xaml.cs file and bind it to LocalNotificationCommand.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="Forms.Mobile.Views.MainPage"
NavigationPage.HasNavigationBar="False">
<StackLayout HorizontalOptions="CenterAndExpand" VerticalOptions="CenterAndExpand">
<StackLayout HorizontalOptions="Center"
Margin="0,10">
<Button Text="Local Notification"
BackgroundColor="#09C"
TextColor="#FFF"
Command="{Binding LocalNotificationCommand}"
WidthRequest="150"/>
</StackLayout>
</StackLayout>
</ContentPage>
In the MainPageVeiwModel.cs, create new command called LocalNotification and intialize in the constructor:
public DelegateCommand LocalNotificationCommand { get; private set; }
public MainPageViewModel(INavigationService navigationService)
: base(navigationService)
{
Title = "Main Page";
LocalNotificationCommand = new DelegateCommand(ShowNotification);
}
After that, create a new folder called Middle ware, in which, we can define all the interfaces that can represent common behaviors between the different operating system ( For example, using localisation). In this case, you will create a new interface called INotificationManager.cs. Define 3 major methods Initialize, ScheduleNotification and ReceiveNotification.
public interface INotificationManager
{
void Initialize();
void ScheduleNotification(string title, string message);
void ReceiveNotification(string title, string message);
}
Create NotificationService
Now, let’s create Services folder in the main project, in which we define all the services and its interfaces that will be user in our ViewModels.
Create Interfaces folder inside Services folder. Now add service file called NotificationService.cs and its interface INotificationService.cs
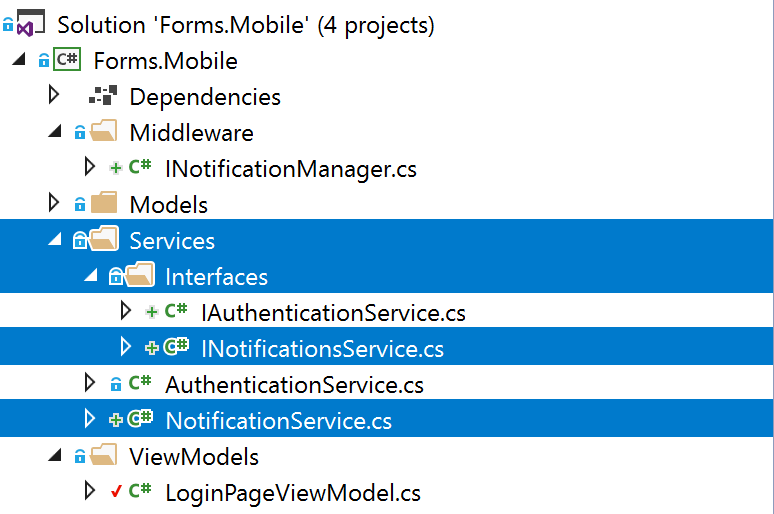
public interface INotificationsService
{
void ShowNotification(string title, string message);
}
public class NotificationsService : INotificationsService
{
private readonly INotificationManager notificationManager;
public NotificationsService()
{
notificationManager = DependencyService.Get<INotificationManager>();
}
public void ShowNotification(string title, string message)
{
notificationManager.ScheduleNotification(title, message);
}
}
We will call notificationManager.ScheduScheduleNotification that will be defined in each project(Android and IOS)
Android NotificationManager
You have to define in Android project the behavior of this notification. That’s why you need to create a new file called AndroidNotificationManager.cs implements the INotificationManager defined in the main project.
Define the properties of default Id and name of the notification channel. From the API 26, the notification doesn’t work without creation of notification channel. Check the official documentation web site.
In the method CreateNotificationChannel, verify if the version of the API bigger then 26 (BuildVersionCodes.O) then create the notification channel and call it in Initialize method.
private void CreateNotificationChannel()
{
notificationManager = (NotificationManager)AndroidApp.Context.GetSystemService(AndroidApp.NotificationService);
// If API >= 26 then we need to create a channel for notification.
if (Build.VERSION.SdkInt >= BuildVersionCodes.O)
{
var channelNameJava = new Java.Lang.String(defaultChannelName);
var channel = new NotificationChannel(defaultChannelId, channelNameJava, NotificationImportance.High)
{
Description = defaultChannelDescription
};
notificationManager.CreateNotificationChannel(channel);
}
channelInitialized = true;
}
Now, let’s define the ScheduleNotification method. We check if the channel was already initialized or not. After that we add the intent that represents witch activity when user tap on the notification. In our case it will be the Main Activity.
Finally Build the notification with the different parameters like the icon to be shown in the notification, the sound to be played and eventually the title and message.
public void ScheduleNotification(string title, string message)
{
if (!channelInitialized)
{
CreateNotificationChannel();
}
Intent intent = new Intent(AndroidApp.Context, typeof(MainActivity));
//Intent deleteIntent = new Intent(AndroidApp.Context, typeof());
intent.PutExtra("title", title);
intent.PutExtra("message", message);
messageId++;
PendingIntent pendingIntent = PendingIntent.GetActivity(AndroidApp.Context, pendingIntendId, intent, PendingIntentFlags.OneShot);
NotificationCompat.Builder builder = new NotificationCompat.Builder(AndroidApp.Context, defaultChannelId)
.SetContentIntent(pendingIntent)
.SetDefaults(NotificationCompat.DefaultAll)
.SetContentTitle(title)
.SetContentText(message)
.SetLargeIcon(BitmapFactory.DecodeResource(AndroidApp.Context.Resources, Resource.Mipmap.ic_launcher))
.SetSmallIcon(Resource.Mipmap.ic_launcher)
.SetAutoCancel(true);
Notification notification = builder.Build();
notificationManager.Notify(messageId, notification);
}
Deploy the application and run it on the emulator you will see something like this:
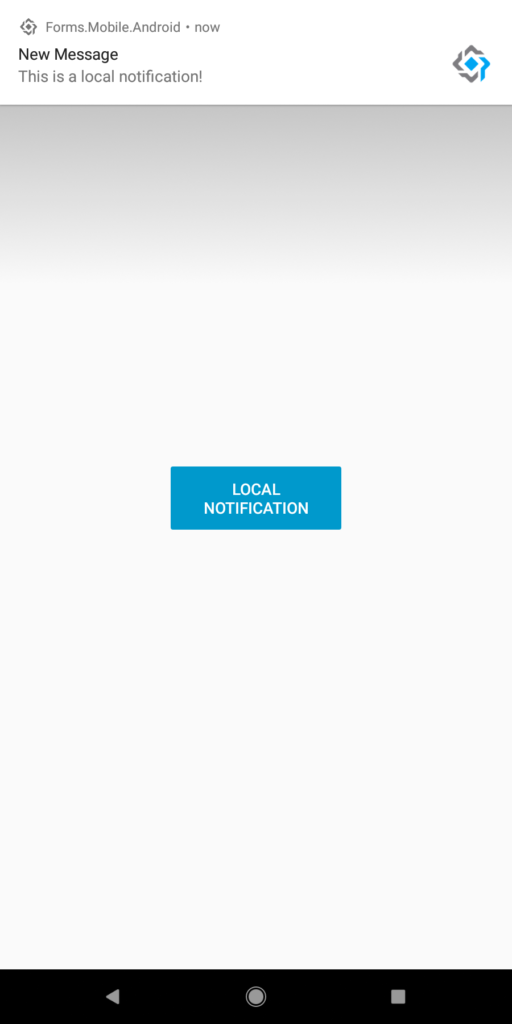
Follow Me For Updates
Subscribe to my YouTube channel or follow me on Twitter or GitHub to be notified when I post new content.